Mutex vs Semaphore: Key Differences and Use Cases
Concurrency control is crucial in multi-threaded programming. Mutexes and semaphores are two common synchronization tools used to manage access to shared resources. Understanding their differences and use cases is essential for building efficient and safe applications.
What is a Mutex?β
A mutex (short for mutual exclusion) acts like a lock you put on a door to make sure only one person (thread or process) can enter at a time. It ensures that only one thread can access a critical section of code, preventing conflicts and data corruption.
Real-World Exampleβ
Imagine a single bathroom in your house. When someone locks the door (acquires the mutex), no one else can enter until the person inside unlocks the door (releases the mutex). This ensures privacy and prevents any interruptions.
-
Purpose: To ensure that only one thread or process can access a shared resource at a time.
-
Ownership: Only the thread that locks the mutex can unlock it. This guarantees that the resource is properly released and not accidentally modified by other threads.
-
Use Case: Protecting critical sections where shared resources like data, variables, or files are accessed. For example, in database transactions or file handling to prevent data corruption.
In the Critical Section Problem, we used a mutex to protect shared data from being accessed simultaneously by multiple threads.
What is a Semaphore?β
A semaphore is like a ticket system at a busy restaurant. It controls how many people (threads or processes) can access a shared resource simultaneously, rather than restricting it to just one.
Real-World Exampleβ
Consider a restaurant with 10 tables. The semaphore starts with a value of 10, representing the available tables. Each time a group of diners enters, they take a ticket (decrement the semaphore). When they leave, they return the ticket (increment the semaphore), freeing up a table for others.
-
Purpose: To manage access to a pool of shared resources, allowing multiple threads to use the resources simultaneously.
-
Ownership: Unlike a mutex, semaphores do not have strict ownership. Any thread can increase (signal) or decrease (wait) the semaphore value, making it more flexible for managing resources.
-
Use Case: Ideal for scenarios where multiple instances of a resource need to be managed, like limiting the number of database connections or controlling thread access to a shared data structure.
Types of Semaphoresβ
-
Binary Semaphore: Acts like a mutex, allowing only one thread to access the resource. The semaphore value can only be 0 or 1.
-
Counting Semaphore: Allows multiple threads to access the resource simultaneously. The semaphore value can be any number, indicating the number of available resources.
Real-world Example: In the Producer-Consumer Problem, a counting semaphore is used to manage access to a shared queue. If the queue is full, producers wait until a consumer removes an item.
Mutex vs Semaphore: Understanding the Differencesβ
Mutex and semaphore serve different purposes, and each has its advantages. Letβs explore scenarios that highlight their unique features.
Priority Inversion with Mutexβ
What is Priority Inversion?β
Priority inversion occurs when a high-priority task is waiting for a lower-priority task to release a resource, and a medium-priority task preempts the lower-priority task. This causes the high-priority task to wait longer than necessary.
Example Scenario:
-
P1: Low priority
-
P2: Medium priority
-
P3: High priority
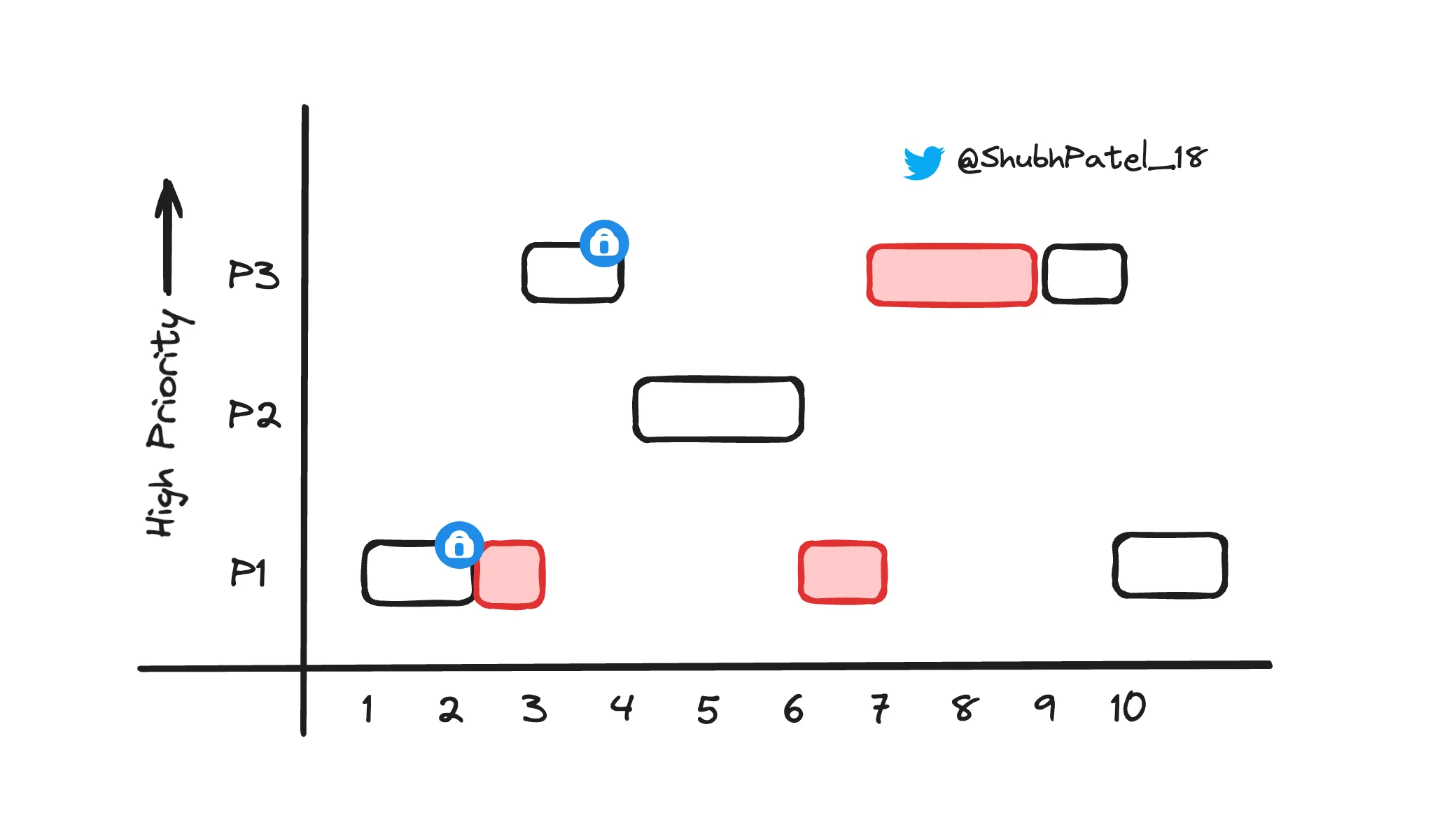
Priority Inversion
-
P1 starts execution and locks a mutex to use a resource.
-
P3 preempts P1 because it has higher priority but is blocked waiting for the mutex held by P1.
-
P2, with medium priority, preempts P1. Now, P1 is delayed, causing P3 to be blocked longer.
-
This scenario causes P3 (high priority) to wait unnecessarily because P2 (medium priority) is running, which is called priority inversion.
Solving Priority Inversion with Priority Inheritanceβ
To address priority inversion, priority inheritance temporarily boosts the priority of the lower-priority task holding the resource to match that of the highest-priority waiting task.
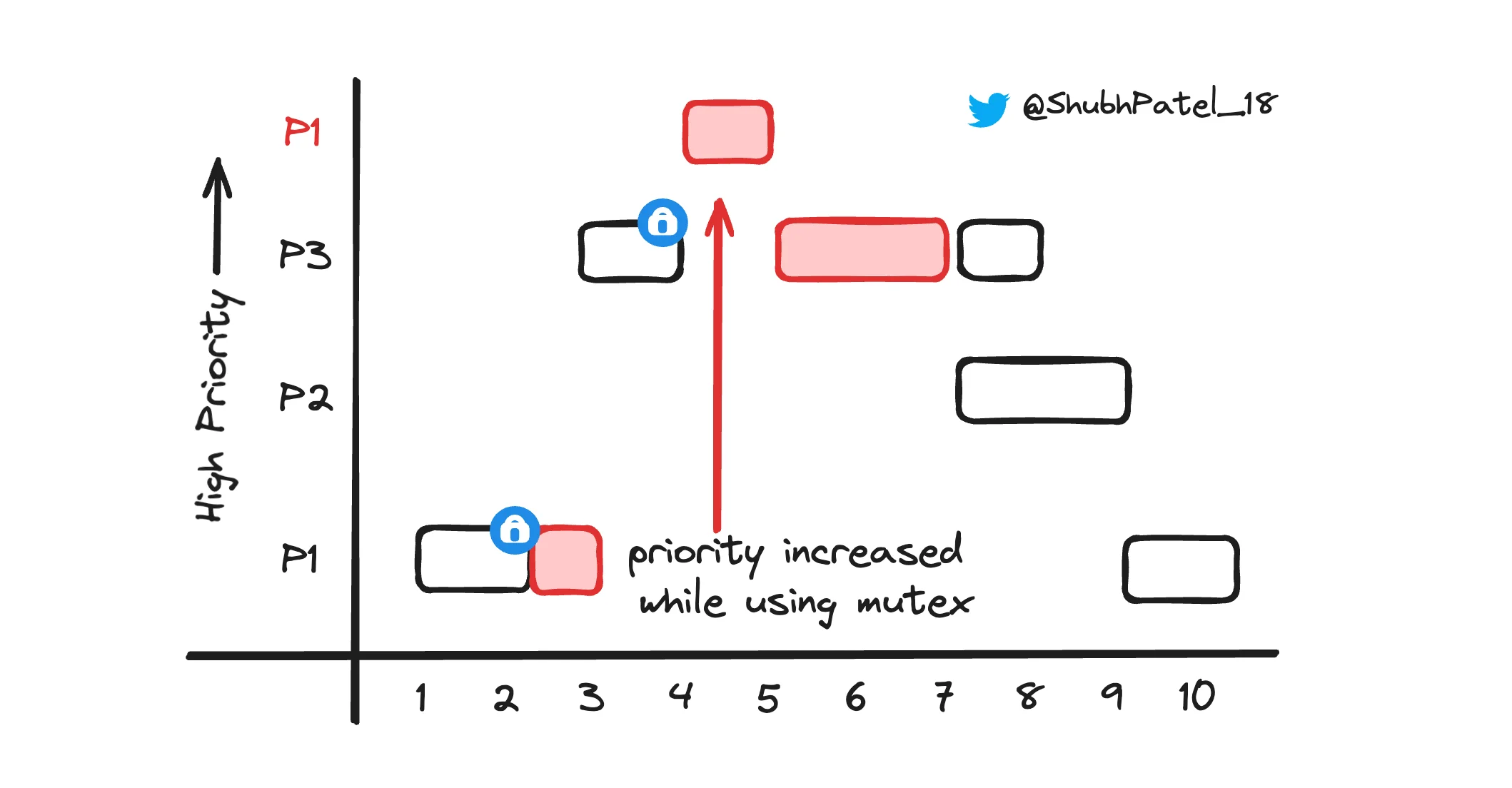
Priority Inheritance
-
P1 starts and acquires the mutex.
-
P3 arrives and wants the mutex but is blocked.
-
The system temporarily boosts P1's priority to match P3's.
-
P1 completes its task quickly because it now has higher priority than P2.
-
P1 releases the mutex, allowing P3 to proceed.
-
P1βs priority is restored to its original level.
Why is this better?
This method prevents high-priority tasks from being delayed by medium-priority tasks, ensuring that critical tasks complete quickly and efficiently.
Interrupt Handling with Semaphoresβ
Interrupt Service Routines (ISRs) are critical in embedded and real-time systems for handling events quickly.
Using Mutex with ISRsβ
Problem: If the main thread locks a mutex and an interrupt occurs, the ISR may need access to the same resource. Mutexes donβt allow ISRs to proceed if they are locked, causing potential deadlocks.
Using Semaphores with ISRsβ
-
Solution: Semaphores allow ISRs to increase the semaphore count, granting temporary access to shared resources.
-
How it works:
-
The semaphore controls access to the resource.
-
When an interrupt occurs, the ISR can increment the semaphore to gain immediate access.
-
After the ISR completes, it decrements the semaphore, releasing the resource.
-
Why Semaphores are Better for ISRs:
-
Flexibility: Semaphores do not have strict ownership rules, so ISRs can access shared resources without waiting.
-
Avoiding Deadlocks: By using semaphores, ISRs can complete their tasks quickly, ensuring efficient interrupt handling.
Conclusionβ
-
Mutex: Best for exclusive access to a resource, ensuring only one thread can access the critical section at a time. Ideal for preventing data corruption when threads need strict control over resources.
-
Semaphore: Best for managing multiple access to a pool of resources, providing flexibility in controlling resource usage. Suitable for scenarios where many threads need access or for interrupt handling in real-time systems.
Understanding when to use a mutex versus a semaphore is essential for building efficient, deadlock-free applications that can handle concurrent operations smoothly.